Local development
Inngest's tooling makes it easy to develop your functions locally with any framework using the Inngest Dev Server.
The Inngest Dev Server is a fully-featured and open-source local version of the Inngest Platform enabling a seamless transition from your local development to feature, staging and production environments.
Get started with your favorite setup:
Inngest Dev Server
Start Inngest locally with a single command.
Development with Docker
Run Inngest locally using Docker or Docker Compose.
Development Flow with Inngest
The Inngest Dev Server provides all the features available in the Inngest Platform, guaranteeing a smooth transition from local dev to production environments.
Developing with Inngest looks as it follows:
- Configure the Inngest SDK in your application
- Connecting the Inngest Dev Server to your local application
- Develop your Inngest Functions with Steps, Flow Control and more
- (Optional) - Configure Preview environments with our Vercel Integration
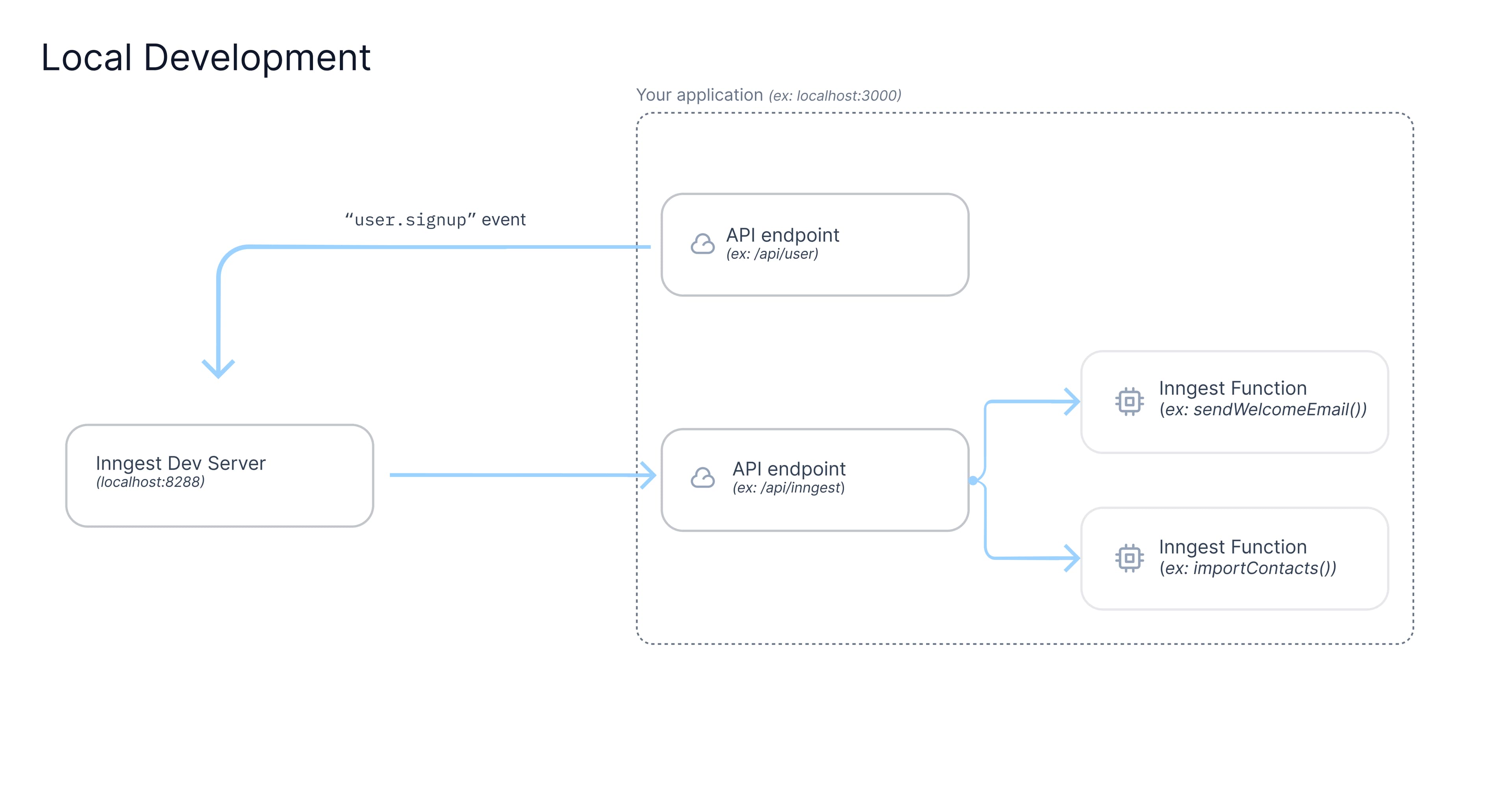
Moving to production environments (preview envs, staging or production)
Deploying your application to preview, staging and production environments does not require any code change:
- Create an Inngest App on the Inngest Platform and configure its Event and Signing Keys on your Cloud.
- Leverage the Inngest Platform to manage and monitor Events and Function Runs
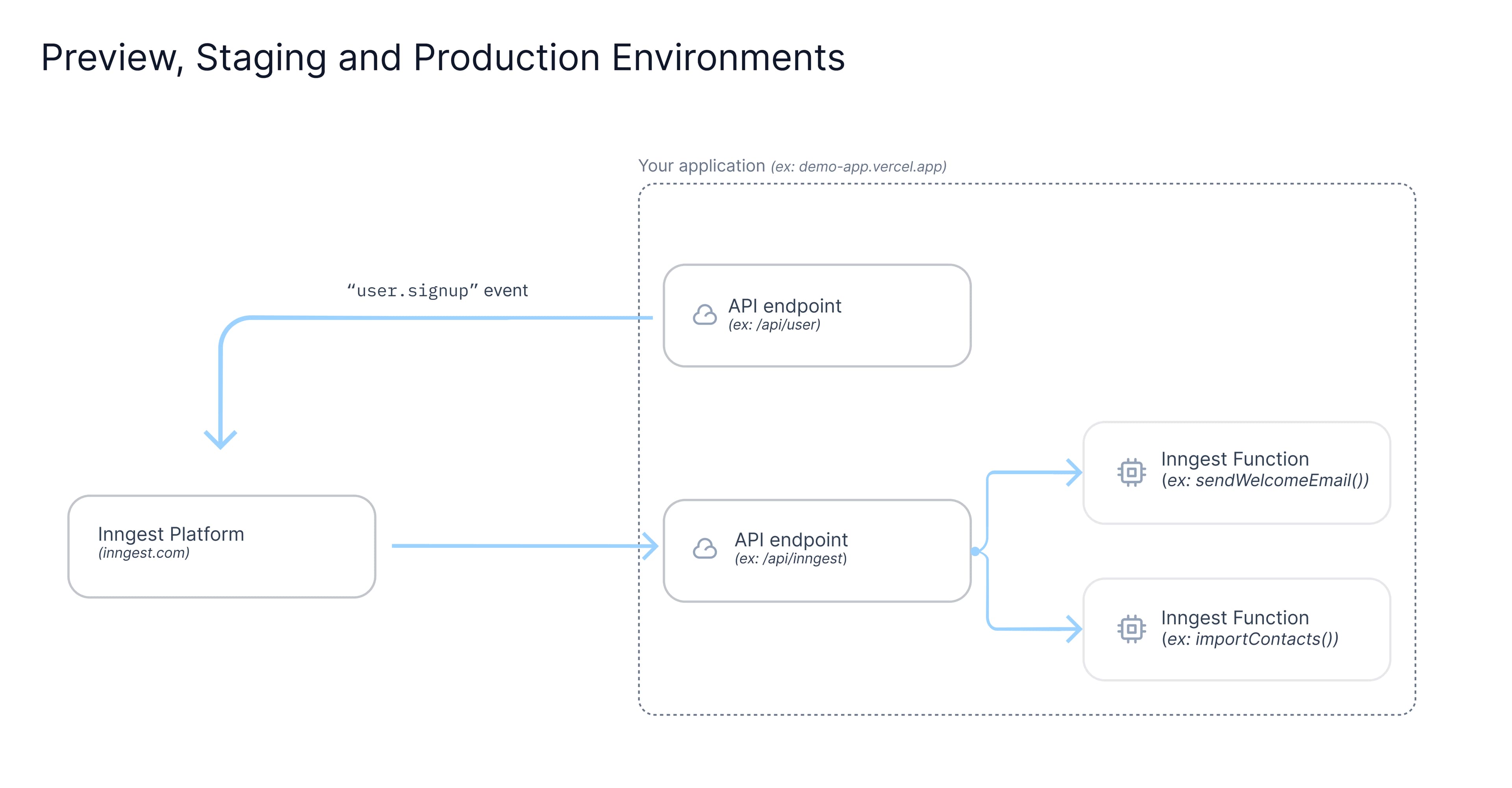
CLI and SDKs
TypeScript SDK
Setup the Inngest SDK in your TypeScript application.
Python SDK
Setup the Inngest SDK in your Python application.
Go SDK
Setup the Inngest SDK in your Go application.
FAQs
Can I run the Inngest Dev Server in production?
The Inngest Dev Server is not designed to be run in production, but you can run it anywhere that you want including testing environments or CI/CD pipelines.
How do I test webhooks locally?
Webhooks configured on the Platform can be sent to the Dev Server.
How do I work with Stripe or Clerk webhooks locally?
External webhooks from Stripe and Clerk must go through a tunnel solution (such as ngrok or localtunnel) to reach the Dev Server.
Are Crons supported locally?
Yes. You can also trigger a function at any time by using the "Invoke" button from the Dev Server Functions list view.
Find more answers in our Discord community.