Debounce
Debounce delays function execution until a series of events are no longer received. This is useful for preventing wasted work when a function might be triggered in quick succession. Use cases for debounce include:
- Preventing wasted work when handling events from user input that may change multiple times in a short time period.
- Delaying processing of noisy webhook events until they are no longer received.
- Ensuring that functions use the latest event within a series of updates (for example, synchronization).
How to configure debounce
export default inngest.createFunction(
{
id: "handle-webhook",
debounce: {
key: "event.data.account_id",
period: "5m",
timeout: "10m",
},
},
{ event: "intercom/company.updated" },
async ({ event, step }) => {
// This function will only be scheduled 5 minutes after events are no longer received with the same
// `event.data.account_id` field.
//
// `event` will be the last event in the series received.
}
);
Configuration reference
period
- The time delay to delay execution. The period begins when the first matching event is received.key
- An optional expression using event data to apply each limit too. Each unique value of thekey
has its own limit, enabling you to rate limit function runs by any particular key, like a user ID.timeout
- Optional. The maximum time that a debounce can be extended before running.
How it works
When a function is triggered, the debounce period
begins. If another event is received that matches the function's trigger, the debounce period
is reset. This continues until no events are received for the debounce period
. Once the period
has passed without any new events, the function is executed using the last event received.
If a timeout
is provided, the function will always run after the timeout
has passed even if new events are received. This ensures that the function does not continue to be debounced indefinitely if events continue to debounce the function.
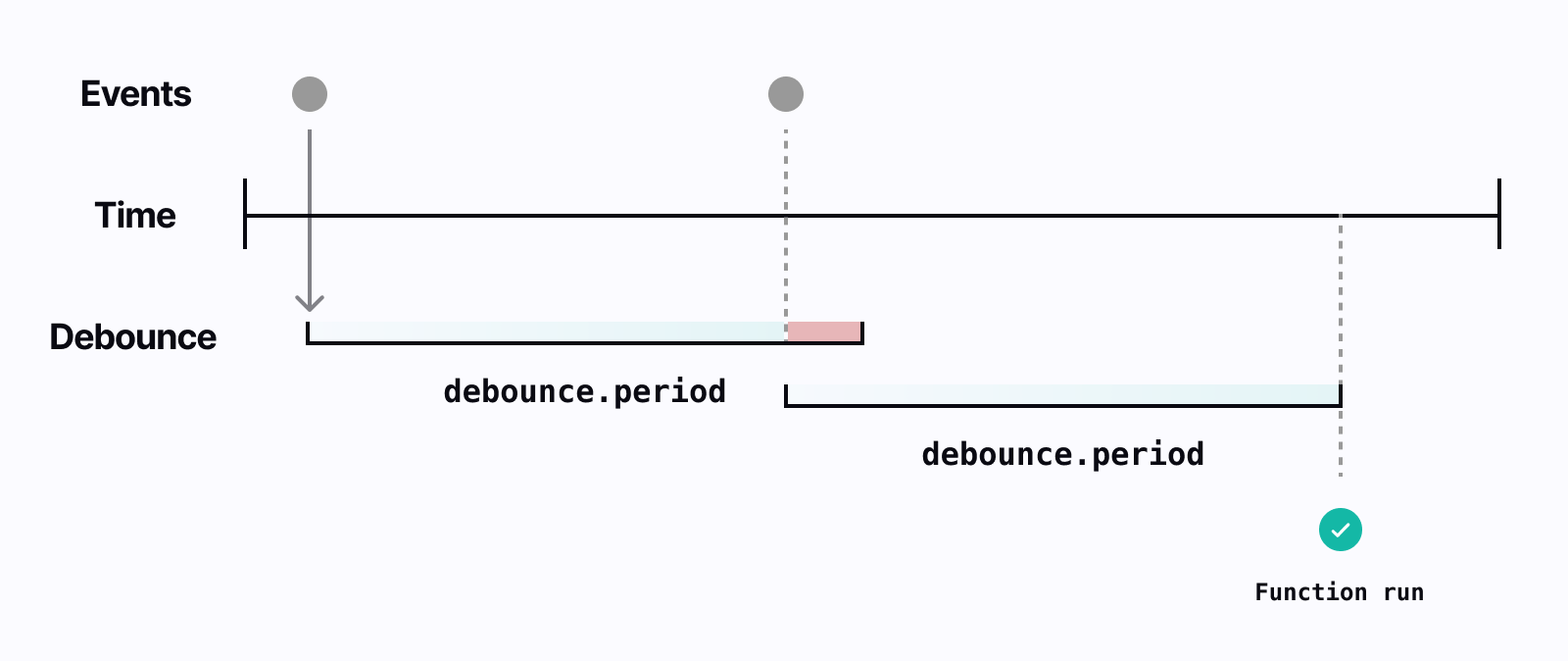
Using a key
When a key
is added, a separate debounce period is applied for each unique value of the key
expression. For example, if your key
is set to event.data.customer_id
, each customer would have their individual debounce period applied to functions run. Read our guide to writing expressions for more information.
Comparison to rate limiting
If you prefer to execute a function for the first event received, consider using rate limiting instead. Rate limiting ensures that a function runs once for each key
the first time an event is received, while debounce uses the last event during a specified period.
Combining with idempotency
Debounce can be combined with idempotency to ensure that once the debounced function has run, it does not run again.
Limitations
- The maximum debounce
period
is 7 days (168 hours). - The minimum debounce
period
is 1 second. - Debounce does not work with batched functions.